While working on website for my sister, I was perturbed that there were no libraries on Clojars for sending email. I was using Leiningen which will download and include all your dependancies for you (so sweet!).
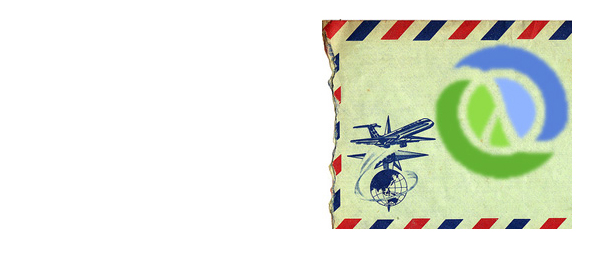
Yet, with the library I found, I had to manually download all the required jars. Call me lazy but being forced to manually download dependancies seems like cruel and unusual punishment these days.
So I solved the problem with MMEmail: Simple Clojure library for sending email, with just one jar!
Installation
Leiningen
(defproject your-project "0.0.0"
:dependencies [[mmemail "1.0.0"]])
Jar File
Can be downloaded at http://clojars.org/repo/mmemail/mmemail/1.0.0/mmemail-1.0.0.jar
Usage
Include the library:
(use 'mmemail.core)
The Easy Way
mmemail.core
is the only include you need. It contains only 2 methods, the fist being send-email. It takes a map that includes all the configuration and email parameters. That’s it.
(send-email {:host "smtp.gmail.com"
:port 465
:ssl true
:user "road@gmail.com"
:password "runner"
:to "joe@acme.com"
:subject "Greetings"
:body "Meep Meep!"})
Most of the parameters are required, but based on your server configuration you might get away without these:
- :ssl
- :password
- :subject
It also accepts the following optional parameters:
- :from (defaults to :user)
- :cc
- :bcc
The recipient parameters (:to, :cc, :bcc) may be a string, for one recipient, or a sequence of strings, for multiple recipients.
The Convenient Way
It can be annoying to pass such a big map into the function, and typically you’ll want to get all the server configuration out of the way. This is where the second function of mmemail.core (create-mailer)
comes into play.
(def my_mailer (create-mailer {:host "smtp.gmail.com"
:port 465
:ssl true
:user "road@gmail.com"
:password "runner"}))
Create-mailer
will return a new function with all the configuration baked in. So in the future, you can send email like so:
(my_mailer {:to "joe@acme.com"
:subject "Greetings"
:body "Meep Meep!"})
The create-mailer
accepts email parameters that will be used as defaults when the generated function is called.
(def my_mailer (create-mailer {:subject "Greetings"
:body "Meep Meep!"
:host "smtp.gmail.com"
:port 465
:ssl true
:user "road@gmail.com"
:password "runner"}))
(my_mailer {:to "joe@acme.com"})
License
Copyright 2010 Micah Martin. All Rights Reserved. MMEmail and all included source files are distributed under terms of the GNU LGPL.